{ your data }
Capture datasets at the application level, fine tune best in class models, and deploy with our optimized inference engine.
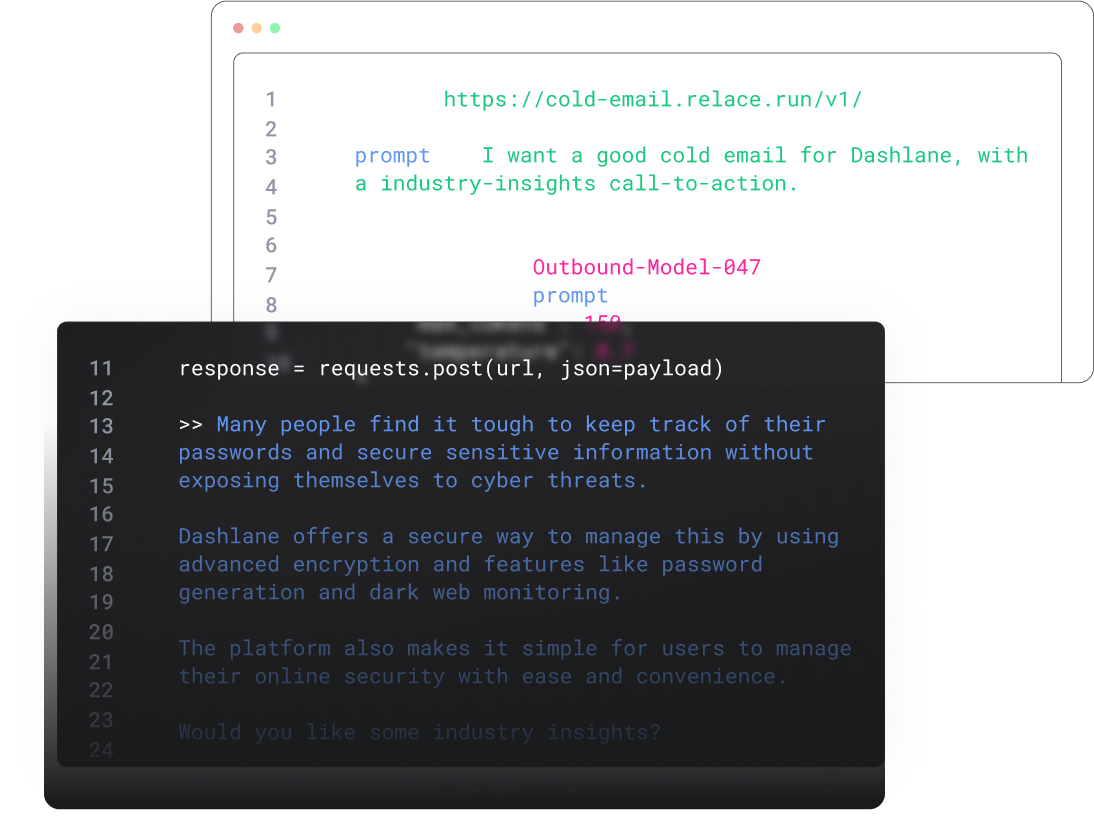
OUR INVESTORS



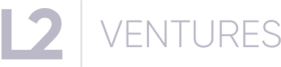
Training
Fine tuning made as simple as possible, but no simpler.
Every use case is different, and some require more attention than others. Use our out-of-the-box training hyperparameters, craft your own, or reach out to our support team of ML engineers for task-specific optimizations.
Evaluation Loss Curve
Enqueued
Startup
Execution
Status
09:34:22 AM
0.0s
-
09:34:19 AM
0.0s
0.0s
09:34:15 AM
0.1s
2.2s
09:34:14 AM
0.0s
1.9s
09:34:12 AM
0.2s
1.7s
09:34:10 AM
0.0s
2.1s
Development
Fastest inference made affordable.
Whether you need a shared SLoRA system, dedicated deployment, or custom built setup on your own VPC, Relace is consistently on the bleeding edge of the cost/latency frontier.
Code
Start capturing data today, and improve your model performance tomorrow
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
from relace import OpenAI
client = OpenAI(
api_key="OPENAI_API_KEY",
relace_api_key="RELACE_API_KEY",
)
messages = [
{
"role": "system",
"content": "..."
},
{
"role": "user",
"content": "..."
}
]
completion = client.chat.completions.create(
model="gpt-4o",
messages=messages,
)
from relace import OpenAI client = OpenAI( api_key= "OPENAI_API_KEY", relace_api_key= "RELACE_API_KEY", ) messages = [ { "role": "system", "content": "..." }, {"role": "user", "content": "..." } ] completion = client.chat.completions.create( model="gpt-4o", messages= messages, )
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
import OpenAI from 'relace';
const client = new OpenAI({
apiKey: "OPENAI_API_KEY",
relaceApiKey: "RELACE_API_KEY"
});
const messages = [
{
role: "system",
content: "..."
},
{
role: "user",
content: "..."
}
];
const completion = await client.chat.completions.create({
model: "gpt-4o",
messages: messages
});
import OpenAI from 'relace'; const client = new OpenAI({ apiKey: "OPENAI_API_KEY", relaceApiKey: "RELACE_API_KEY" }); const messages = [ { role: "system", content: "..." }, { role: "user", content: "..." } ]; const completion = await client.chat.completions.create({ model: "gpt-4o", messages: messages });
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
curl https://api.openai.com/v1/chat/completions \ -H "Content-Type: application/json" \ -H "Authorization: Bearer OPENAI_API_KEY" \ -H "X-Relace-Key: RELACE_API_KEY" \ -d '{ "model": "gpt-4o", "messages": [ { "role": "system", "content": "..." }, { "role": "user", "content": "..." } ] }'
curl https://api.openai.com/v1/chat/completions \
-H "Content-Type: application/json" \
-H "Authorization: Bearer OPENAI_API_KEY" \
-H "X-Relace-Key: RELACE_API_KEY" \
-d '{
"model": "gpt-4o",
"messages": [
{
"role": "system",
"content": "..."
},
{
"role": "user",
"content": "..."
}
]
}'
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
from relace import OpenAI client = OpenAI( api_key= "OPENAI_API_KEY", relace_api_key= "RELACE_API_KEY", ) messages = [ { "role": "system", "content": "..." }, {"role": "user", "content": "..." } ] completion = client.chat.completions.create( model="gpt-4o", messages= messages, )
const response = await fetch('https://api.openai.com/v1/chat/completions', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
'Authorization': `Bearer ${OPENAI_API_KEY}`,
'X-Relace-Key': RELACE_API_KEY
},
body: JSON.stringify({
model: 'gpt-4o',
messages: [
{
role: 'system',
content: '...'
},
{
role: 'user',
content: '...'
}
]
})
});
const data = await response.json();
const response = await fetch('https://api.openai.com/v1/chat/completions', { method: 'POST', headers: { 'Content-Type': 'application/json', 'Authorization': `Bearer ${OPENAI_API_KEY}`, 'X-Relace-Key': RELACE_API_KEY }, body: JSON.stringify({ model: 'gpt-4o', messages: [ { role: 'system', content: '...' }, { role: 'user', content: '...' } ] }) }); const data = await response.json();